C Programming/Basic Output/Example 1
This example will show you how to use escape sequences and do text formatting.
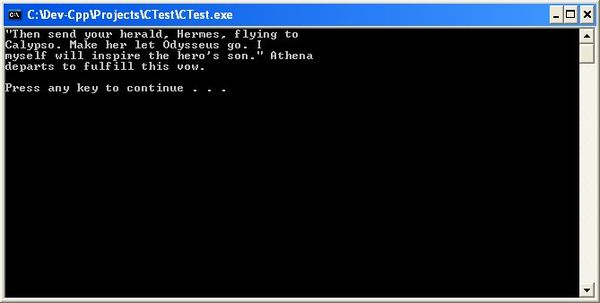
Here is the source code:
#include <stdio.h>
int main(void)
{
printf("\"Then send your herald, Hermes, flying to\n");
printf("Calypso. Make her let Odysseus go. I\n");
printf("myself will inspire the hero\'s son.\" Athena\n");
printf("departs to fulfill this vow.\n\n");
getchar();
return 0;
}
If you will notice, at the end of every string in the printf()
functions there is a \n, this is so a new line starts for every line.
In the first prinf()
call there is a \" escape sequence. This is so the double quote will be printed to the console and not be seen by the compiler as something terminating or initiating a string. Same goes for the single quote in the third call of printf()
.
If you intend to print unformatted strings, you can use the function puts
to supply the same output:
#include <stdio.h>
int main(void)
{
puts("\"Then send your herald, Hermes, flying to");
puts("Calypso. Make her let Odysseus go. I");
puts("myself will inspire the hero\'s son.\" Athena");
puts("departs to fulfill this vow.\n");
getchar();
return 0;
}
puts
always appends a newline to whatever it writes, so in this example there is no need for the newline escape sequences, except for the very last one where we require two (one supplied by puts
, one supplied by our string).